Create a Component
This tutorial assumes you are using windows and can run the Universal Windows Version of VertiGIS Studio Mobile.
If you can't solve your use case by editing the app layout and config using the built-in components, then it may be time to learn how to extend VertiGIS Studio Mobile with custom components. With custom components, you can create completely configurable UI and behavior, interact with other components and built-in services, and more.
Prerequisites
Check out and setup the VertiGIS Studio Mobile SDK Quickstart project.
Basic Component
Create a new file components/CustomComponent.cs
in your project.
In the file, add a new component class CustomComponent
and register it with VertiGIS Studio Mobile using an assembly attribute.
using App1;
using App1.Components;
using System.Xml.Linq;
using VertiGIS.Mobile.Composition.Layout;
[assembly: Component(typeof(CustomComponent), "custom-component", XmlNamespace = XmlNamespaces.App1Namespace)]
namespace App1.Components
{
class CustomComponent : ComponentBase
{
protected override VisualElement Create(XNode node)
{
return new Label() { Text = "My Custom Component" };
}
}
}
Add the Component to your Layout
You can now add the component to your app layout to see it in the application.
- Add the
XmlNamespaces.App1Namespace
namespace with the aliascustom
to your xml namespaces. - Place your custom component in a taskbar panel.
- Layout
- App Config
- User Interface
<?xml version="1.0" encoding="utf-8" ?>
<layout
xmlns="https://geocortex.com/layout/v1"
xmlns:gxm="https://geocortex.com/layout/mobile/v1"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://geocortex.com/layout/v1 ../../ViewerSpec/layout/layout-mobile.xsd"
xmlns:custom="https://your.org/layout/app1">
<gxm:taskbar>
<map slot="main"/>
<panel>
<custom:custom-component icon="info"/>
</panel>
</gxm:taskbar>
</layout>
{
"$schema": "..\\..\\ViewerFramework\\app-config\\mobile\\mobile-app-config.schema.json",
"schemaVersion": "1.0",
"items": [
{
"id": "desktop-layout",
"$type": "layout",
"url": "resource://layout-large.xml",
"tags": ["medium", "large"]
}
]
}
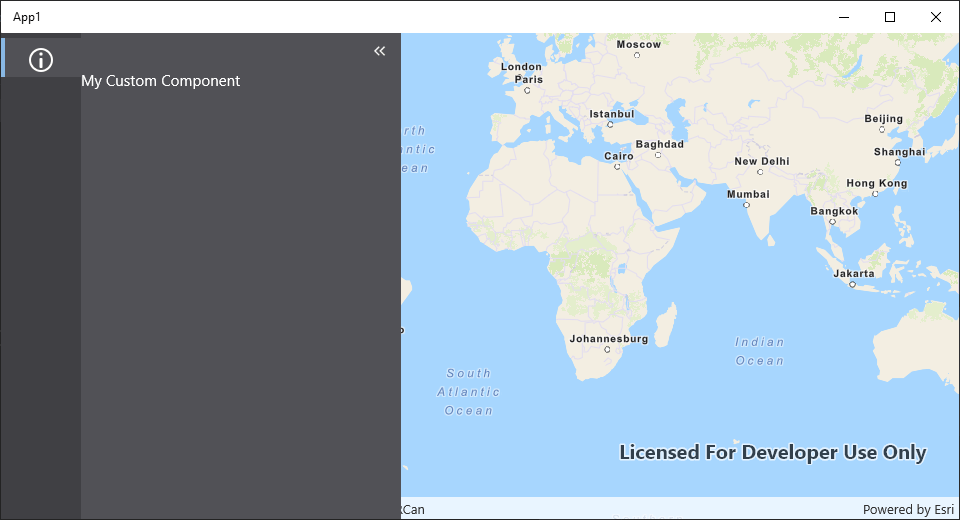
You've now accomplished the basics of extending VertiGIS Studio Mobile with a custom component. With custom components, you can create completely new user interfaces and run completely custom logic in your VertiGIS Studio Mobile application.
Relevant SDK Samples
Check out the relevant VertiGIS Studio Mobile SDK Samples:
Next Steps
Create a Custom Service
Learn how to create a custom service with the SDK
Check out the Component Reference
Take a deep dive into components in the VertiGIS Studio Mobile SDK
Create a Component with a Complex UI
Build a custom, interactive component with a UI defined in XAML
Create a Component that Consumes App Config
Use app configuration to create easily customizable components