Pull Component Data from App Config
A component can optionally participate in the app config. This allows for the component's behavior and initial state to be easily modified without having to deploy custom code. Different instances of the same component type can reference different app config items, allowing for multiple components in the same application with different behavior.
Prerequisites
Check out and setup the VertiGIS Studio Mobile SDK Quickstart project.
Setup a Basic Component
Copy the following code into your project to setup a basic component without app config.
If you want to learn more about how this component was created, check out the custom component tutorial
- Layout
- Component
- View
- Code Behind
<?xml version="1.0" encoding="utf-8" ?>
<layout
xmlns="https://geocortex.com/layout/v1"
xmlns:gxm="https://geocortex.com/layout/mobile/v1"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://geocortex.com/layout/v1 ../../ViewerSpec/layout/layout-mobile.xsd"
xmlns:custom="https://your.org/layout/app1">
<gxm:taskbar>
<!--Taskbar / custom component-->
<panel>
<custom:custom-component icon="info"/>
</panel>
<map slot="main"/>
</gxm:taskbar>
</layout>
using App1;
using App1.Components;
using VertiGIS.Mobile.Composition.Layout;
using System.Xml.Linq;
[assembly: Component(typeof(CustomComponent), "custom-component", XmlNamespace = XmlNamespaces.App1Namespace)]
namespace App1.Components
{
class CustomComponent : ComponentBase
{
protected override VisualElement Create(XNode node)
{
return new CustomComponentView();
}
}
}
<?xml version="1.0" encoding="UTF-8"?>
<ContentView xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
x:Class="App1.Components.CustomComponentView">
<ContentView.Content>
<Label Text="Hello world!" />
</ContentView.Content>
</ContentView>
using App1.Components;
using VertiGIS.Mobile.Composition;
[assembly: View(typeof(CustomComponentView))]
namespace App1.Components
{
[XamlCompilation(XamlCompilationOptions.Compile)]
public partial class CustomComponentView : ContentView
{
public CustomComponentView()
{
InitializeComponent();
}
}
}
Create a Skeleton App Config Definition
Create a new file CustomComponentConfiguration.cs
. This class defines a new app item type custom-component-config
. This app item type will be used as configuration for the CustomComponent
.
using App1.Configuration;
using VertiGIS.ArcGISExtensions;
using VertiGIS.Mobile.Infrastructure.App;
using System;
[assembly: AppItem(CustomComponentConfiguration.ConfigItemtype, typeof(CustomComponentConfiguration))]
namespace App1.Configuration
{
public class CustomComponentConfiguration : VisualAppItem
{
public const string ConfigItemtype = "custom-component-config";
public string ConfigTitle { get; private set; }
public string ConfigDescription { get; private set; }
public CustomComponentConfiguration()
: this(Guid.NewGuid().ToString())
{
}
public CustomComponentConfiguration(string id)
: this(new Properties { ["id"] = id })
{
}
public CustomComponentConfiguration(Properties properties) :
base(properties, ConfigItemtype)
{
// properties is an object corresponding to the config defined in the app.json
if (properties.TryGetValue("title", out var title))
{
ConfigTitle = title as string ?? "Default Title";
}
if (properties.TryGetValue("description", out var text))
{
ConfigDescription = text as string ?? "Default description.";
}
}
public override object CreateDefault()
{
return new CustomComponentConfiguration();
}
}
}
Consume the Configuration in the Component
Components that consume configuration should extend AppItemComponentBase<ConfigurationClassType>
. This forces them to have a constructor which takes in an IAppItem
resolver for the specific configuration type. This resolver can then be called in the DoInitializeAsync
method to retrieve the app config values. They should also register with Autofac using the AppItemComponent
assembly attribute.
- Component
- View
- Code Behind
- View Model
using App1;
using App1.Components;
using App1.Configuration;
using VertiGIS.Mobile.Infrastructure.App;
using VertiGIS.Mobile.Infrastructure.Layout;
using System.Threading.Tasks;
using System.Xml.Linq;
[assembly: AppItemComponent(typeof(CustomComponent), "custom-component", CustomComponentConfiguration.ConfigItemtype, XmlNamespace = XmlNamespaces.App1Namespace)]
namespace App1.Components
{
class CustomComponent : AppItemComponentBase<CustomComponentConfiguration>
{
private CustomComponentViewModel _viewModel;
IAppItem<CustomComponentConfiguration> _appItemResolver;
public CustomComponent(IAppItem<CustomComponentConfiguration> itemResolver)
: base(itemResolver)
{
_appItemResolver = itemResolver;
_viewModel = new CustomComponentViewModel();
}
protected override VisualElement Create(XNode node)
{
return new CustomComponentView()
{
BindingContext = _viewModel
};
}
protected override async Task DoInitializeAsync()
{
var item = await _appItemResolver.ResolveAsync();
_viewModel.Title = item.ConfigTitle;
_viewModel.Description = item.ConfigDescription;
}
}
}
<?xml version="1.0" encoding="UTF-8"?>
<ContentView xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
x:Class="App1.Components.CustomComponentView">
<ContentView.Content>
<VerticalStackLayout>
<Label FontSize="Title" Text="{Binding Title}" />
<Label Text="{Binding Description}" />
</VerticalStackLayout>
</ContentView.Content>
</ContentView>
using App1.Components;
using VertiGIS.Mobile.Composition;
[assembly: View(typeof(CustomComponentView))]
namespace App1.Components
{
[XamlCompilation(XamlCompilationOptions.Compile)]
public partial class CustomComponentView : ContentView
{
public CustomComponentView()
{
InitializeComponent();
}
}
}
using App1.Components;
using VertiGIS.Mobile.Composition;
using VertiGIS.Mobile.Composition.Views;
[assembly: ViewModel(typeof(CustomComponentViewModel))]
namespace App1.Components
{
public class CustomComponentViewModel : NotifyPropertyBase
{
private string _title;
private string _description;
public string Title
{
get => _title;
set => SetProperty(ref _title, value);
}
public string Description
{
get => _description;
set => SetProperty(ref _description, value);
}
}
}
Define Configuration Values
At this point, the component is hooked up to configuration, and configuration values can be defined and consumed.
App Config is defined in the app JSON and linked to the component through the config
attribute in layout, just like any other component.
- Layout
- App Config
- User Interface
<?xml version="1.0" encoding="utf-8" ?>
<layout
xmlns="https://geocortex.com/layout/v1"
xmlns:gxm="https://geocortex.com/layout/mobile/v1"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://geocortex.com/layout/v1 ../../ViewerSpec/layout/layout-mobile.xsd"
xmlns:custom="https://your.org/layout/app1">
<gxm:taskbar>
<!--Taskbar / custom component-->
<panel>
<custom:custom-component icon="warning" config="custom-component-config"/>
</panel>
<map slot="main"/>
</gxm:taskbar>
</layout>
{
"$schema": "..\\..\\ViewerFramework\\app-config\\mobile\\mobile-app-config.schema.json",
"schemaVersion": "1.0",
"items": [
{
"$type": "custom-component-config",
"id": "custom-component-config",
"title": "Title from Config",
"description": "This description came from config."
},
{
"$type": "layout",
"id": "desktop-layout",
"url": "resource://layout-large.xml",
"tags": ["mobile", "large"]
}
]
}
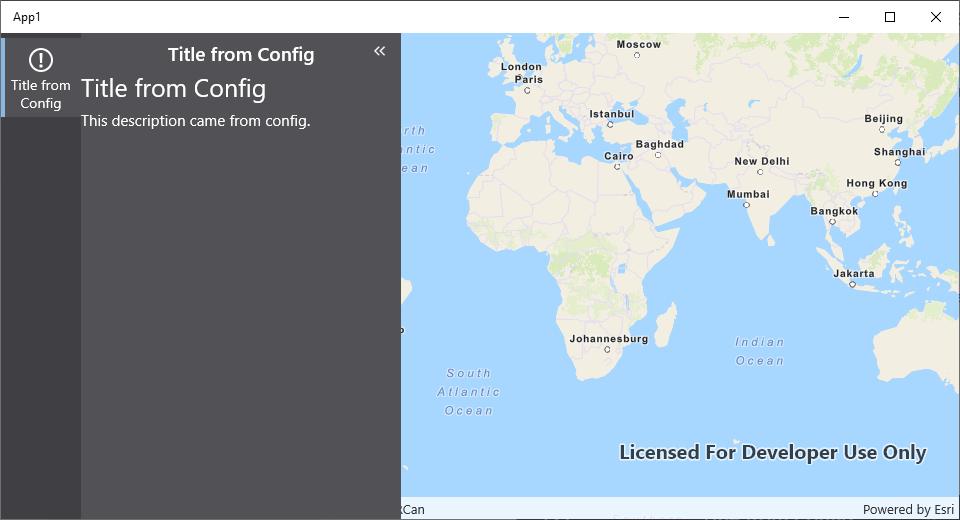
Relevant SDK Sample
Check out the relevant VertiGIS Studio Mobile SDK Sample:
Next Steps
Component Reference
Learn more about components in VertiGIS Studio Mobile
Component and Service Interactions
Learn how components and services interact in VertiGIS Studio Mobile
Implement a Custom Service
Learn how to implement a custom service using the VertiGIS Studio Mobile SDK