Translate a Component's Text
Existing language strings for an application can be customized for any language in the VertiGIS Studio Web Designer. To provide a complete translation in an entirely new locale for VertiGIS Studio Web, please contact us.
Custom components often provide user facing UI with some degree of text. You may want to define their text as language strings for multiple reasons.
- It allows the text be to translated or changed without a corresponding change in the component code.
- It allows the language strings to be shared across different components.
- It consolidates the language strings and makes them easier to locate.
This article will cover creating a german and english translation for a custom component using language strings.
Prerequisites
- Download and setup the VertiGIS Studio Web SDK.
- Check out the deployment instructions to learn more about deploying custom code to a VertiGIS Studio Web App.
Create a Boilerplate Component
First, we will start off with a component that uses a plain hard-coded text string in English.
Learn more about how to build custom components.
- Component
- Model
- Component Index
- Registration
- Layout
- App Config
import React from "react";
import { LayoutElement } from "@vertigis/web/components";
import Typography from "@vertigis/web/ui/Typography";
export default function TranslatableText(props) {
return (
<LayoutElement
{...props}
style={{ backgroundColor: "white" }}
>
<Typography variant="h1">
This text will be translated.
</Typography>
</LayoutElement>
);
}
import {
ComponentModelBase,
serializable,
} from "@vertigis/web/models";
@serializable
class TranslatableTextModel extends ComponentModelBase {}
export default TranslatableTextModel;
export { default } from "./TranslatableText";
export { default as TranslatableTextModel } from "./TranslatableTextModel";
import { LibraryRegistry } from "@vertigis/web/config";
import TranslatableText, {
TranslatableTextModel,
} from "./components/TranslatableText";
export default function (registry: LibraryRegistry) {
registry.registerComponent({
name: "translatable-text",
namespace: "your.custom.namespace",
getComponentType: () => TranslatableText,
itemType: "translatable-text",
title: "Translatable Text",
});
registry.registerModel({
getModel: (config) => new TranslatableTextModel(config),
itemType: "translatable-text",
});
}
<?xml version="1.0" encoding="UTF-8"?>
<layout xmlns="https://geocortex.com/layout/v1" xmlns:custom="your.custom.namespace">
<map>
<custom:translatable-text slot="top-center" width="15" height="9" padding="0.5"/>
</map>
</layout>
{
"schemaVersion": "1.0",
"items": []
}
Creating the Language Resources
Next, we are going to create the language resources for English and German.
- English
- German
{
"language-translatable-text-content": "I will be translated."
}
{
"language-translatable-text-content": "Dieser Text wird übersetzt."
}
Register the Language Resources
Next we need to register the language resources with VertiGIS Studio Web.
import { LibraryRegistry } from "@vertigis/web/config";
import TranslatableText, {
TranslatableTextModel,
} from "./components/TranslatableText";
import enJson from "./en.json";
import deJson from "./de.json";
export default function (registry: LibraryRegistry) {
registry.registerComponent({
name: "translatable-text",
namespace: "your.custom.namespace",
getComponentType: () => TranslatableText,
itemType: "translatable-text",
title: "Translatable Text",
});
registry.registerModel({
getModel: (config) => new TranslatableTextModel(config),
itemType: "translatable-text",
});
registry.registerLanguageResources({
locale: "en",
getValues: () => enJson,
});
registry.registerLanguageResources({
locale: "de",
getValues: () => deJson,
});
}
Use the Language Strings
Now that they have been registered, we can use the strings in our boilerplate component from earlier. VertiGIS Studio Web layout components, like <Typography>
, will automatically detect and translate language keys like language-translatable-text-content
in props and child content.
If you need to manually translate a language key, you can use the useContext
react hook with the UIContext to access the translate
function. Then you can pass the language key language-translatable-text-content
you defined in the language resources files to the translate
function and your text will be translated into whichever locale is most appropriate.
To test out a translation, add the URL parameter locale=<language>
to your local server.
For example, http://localhost:3000/?locale=de
- Component
- English UI
- German UI
import React, { useContext } from "react";
import { LayoutElement } from "@vertigis/web/components";
import { UIContext } from "@vertigis/web/ui";
import Typography from "@vertigis/web/ui/Typography";
export default function TranslatableText(props) {
const { translate } = useContext(UIContext);
return (
<LayoutElement
{...props}
style={{ backgroundColor: "white" }}
>
<Typography
variant="h1"
text="language-translatable-text-content"
/>
<h1>{translate("language-translatable-text-content")}</h1>
</LayoutElement>
);
}
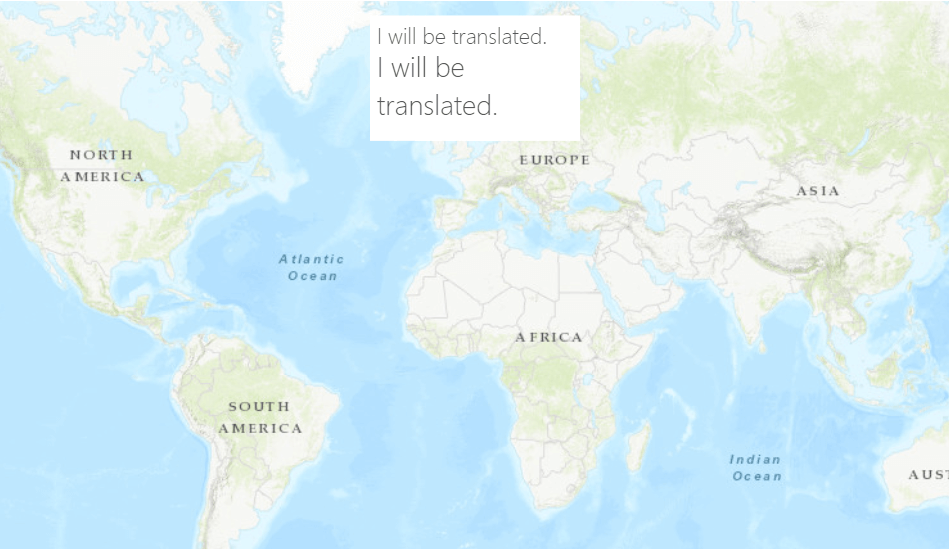
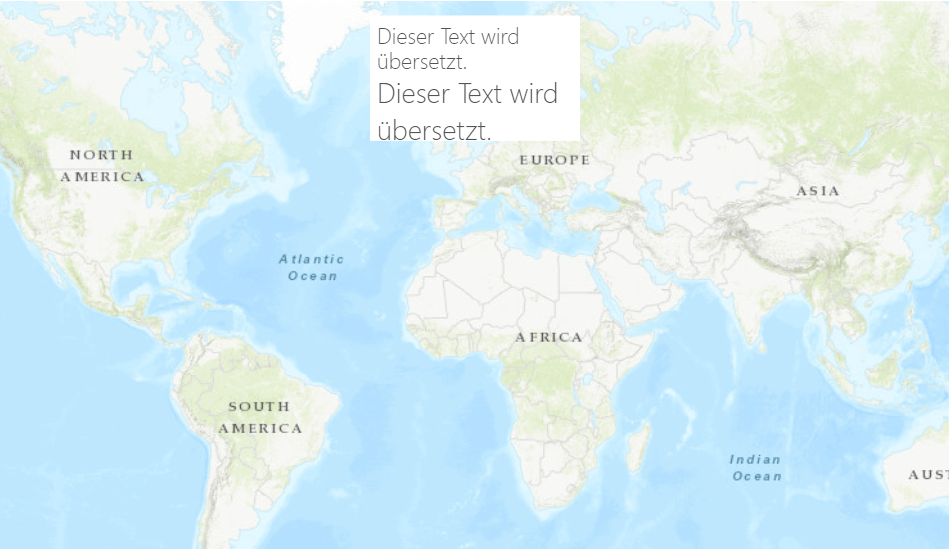
Live Sample
Check out a live SDK sample that demonstrates how to internationalize your application to be supported by multiple locales.
Next Steps
Check out the Language String Reference
Learn more about creating and registering language strings