Create a Component
The VertiGIS Studio Web SDK project template includes an example of a custom component following our best practices. Creating a new custom component involves a few important steps:
- Create the component source file
- Create the component model
- Register the component and component model in your library registration file
Create the Component
Create the component source file in a new folder within the src/components
folder. For example to create a new component called ExampleComponent
, create a new file called ExampleComponent.tsx
in src/components/ExampleComponent
with the following content:
import {
LayoutElement,
LayoutElementProperties,
} from "@vertigis/web/components";
import React from "react";
// We will create this model in the next step.
import ExampleComponentModel from "./ExampleComponentModel";
const ExampleComponent = (
props: LayoutElementProperties<ExampleComponentModel>
) => {
const { model } = props;
return (
<LayoutElement {...props}>
<p>{model.exampleValue}</p>
</LayoutElement>
);
};
export default ExampleComponent;
Create the Component Model
Next we need to create the component model that will be bound to the component we just created. This model will function as the underlying data source for the component.
It's best practice to use the model to define configurable or persistent state, and use the React state hooks for UI specific transient state, like an active selection.
Create a new file called ExampleComponentModel.ts
in src/components/ExampleComponent
:
import {
ComponentModelBase,
ComponentModelProperties,
serializable,
PropertyDefs,
} from "@vertigis/web/models";
interface ExampleComponentModelProperties
extends ComponentModelProperties {
exampleValue?: string;
}
// The serializable decorator marks this class as being serializable
// so it can be serialized to/from the app.json configuration of
// your app.
@serializable
export default class ExampleComponentModel extends ComponentModelBase<ExampleComponentModelProperties> {
exampleValue: string;
// This method defines how the model will be serialized and deserialized into
// an app item. We override it to include our new property `exampleValue`.
protected _getSerializableProperties(): PropertyDefs<ExampleComponentModelProperties> {
const props = super._getSerializableProperties();
return {
...props,
exampleValue: {
serializeModes: ["initial"],
default: "Default Value",
},
};
}
}
Register the Component
Finally we need to register the component and component model with the VertiGIS Studio Web component registry so that it is aware of your new component.
To simplify the module imports, we'll make a new file in the ExampleComponent
folder called index.ts
. Note that this file is for convenience, and is not required:
export { default } from "./ExampleComponent";
export { default as ExampleComponentModel } from "./ExampleComponentModel";
Now register your component and component model with the component registry by modifying the src/index.ts
file:
import { LibraryRegistry } from "@vertigis/web/config";
import ExampleComponent, {
ExampleComponentModel,
} from "./components/ExampleComponent";
// This namespace is generated when you create your project
// and will be unique to your library.
const LAYOUT_NAMESPACE = "custom.abc123";
export default function (registry: LibraryRegistry) {
// ... other item registrations
registry.registerComponent({
name: "example",
namespace: LAYOUT_NAMESPACE,
getComponentType: () => ExampleComponent,
itemType: "example-model",
title: "Example Component",
});
registry.registerModel({
getModel: (config) => new ExampleComponentModel(config),
itemType: "example-model",
});
}
Add the Component to your Layout
You can now add the component to your layout.xml
file and run the development server to see it in use.
- Layout
- UI
<?xml version="1.0" encoding="UTF-8"?>
<layout xmlns="https://geocortex.com/layout/v1" xmlns:custom="custom.abc123">
<custom:example margin="3"/>
</layout>
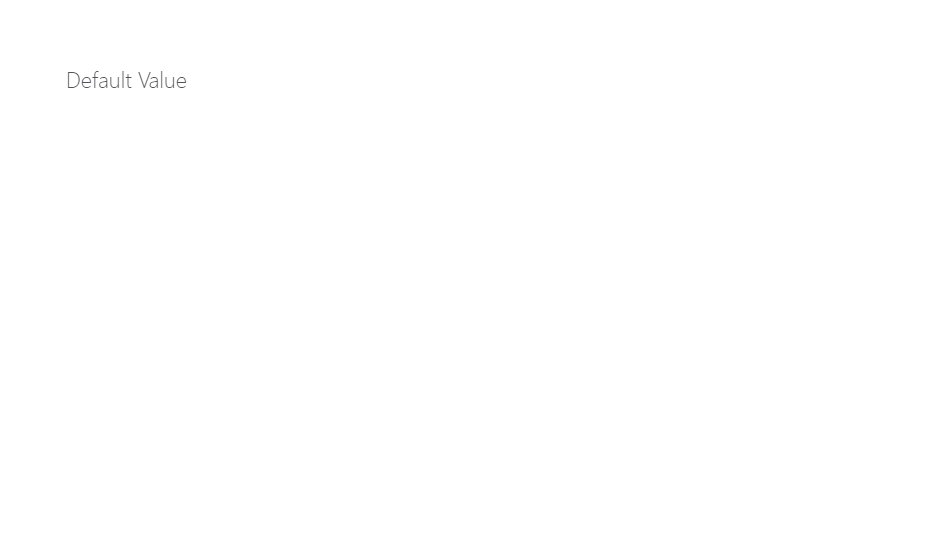
Next Steps
Check out the Component Reference
Take a deep dive into components in the VertiGIS Studio Web SDK
Deploy your Component
Learn how to deploy your custom component.
Create a Component with a Complex UI
Follow along with a more in depth component example
Create a Component that Consumes App Config
Learn more about writing components that consume configuration values.