Implement a Star Rating Custom Form Element using a Third Party Library
Implementing a custom form element allows you to augment the existing form elements that come with VertiGIS Studio Workflow.
This article will walk you through creating a form element that uses the react-star-ratings component to allow the user to select up to five stars.
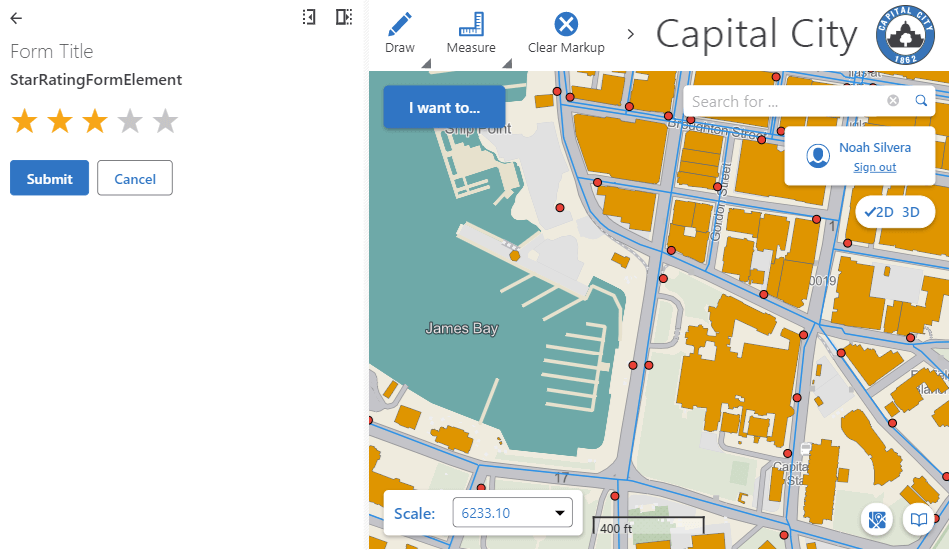
Prerequisites
Follow the instructions in the Web Applications SDK page to set up your development environment.
A working knowledge of TypeScript is recommended before extending Workflow for web-based hosts.
Overview
Implementing a star rating custom form element for web applications using a third party library consists of the following steps:
- Adding third party library dependencies to your project.
- Creating the custom form element.
- Implementing the custom form element using the third party library.
Adding Dependencies
- Run
npm install react-star-ratings
in the terminal to install the third party react-star-ratings component.
Set up Custom Form Element Skeleton
To create a new form element:
- Open the Workflow activity SDK in Visual Studio Code.
- Run
npm run generate
in the terminal. - When prompted, select
Form Element
. - Enter the name of the form element you would like to create and press
Enter
. For example,StarRating
. - Open the newly created
src/elements/StarRating.tsx
file.
Implement the Custom Form Element
Modify the skeleton form element implementation in src/elements/StarRating.tsx
to match the following example.
import * as React from "react";
import StarRatings from "react-star-ratings";
import {
FormElementProps,
FormElementRegistration,
} from "@vertigis/workflow";
interface StarRatingProps extends FormElementProps<number> {}
/**
* A simple React Component that displays a star rating input.
* @param props The props that will be provided by the Workflow runtime.
*/
function StarRating(props: StarRatingProps): React.ReactElement {
const { setValue, value } = props;
// Update the form state when the rating value changes.
const handleRatingChange = (newRating: number) => {
setValue(newRating);
};
return (
<StarRatings
changeRating={handleRatingChange}
rating={value}
starDimension="1.5em"
starHoverColor="#ffb400"
starRatedColor="#ffb400"
/>
);
}
const StarRatingElementRegistration: FormElementRegistration<StarRatingProps> =
{
component: StarRating,
getInitialProperties: () => ({ value: 0 }),
id: "StarRating",
};
export default StarRatingElementRegistration;
Deploy the Form Element
Follow the instructions to build and deploy the activity pack.
Test the Form Element
Once your activity pack is hosted and registered, your StarRating
custom form element should appear in the form element toolbox in VertiGIS Studio Workflow Designer alongside the built-in form elements.
To use your custom form element in a workflow:
- Select the
Display Form
activity that you want to include your form element in. - Locate the
StarRating
form element in the toolbox and drag it onto the form. - Alternatively:
- Add a
Custom
form element to the form. - Set the
Custom Type
property of theCustom
form element toStarRating
.
- Add a
- Test your workflow.