Implement a QR Code Custom Form Element using a Third Party Library
Implementing a custom form element allows you to augment the existing form elements that come with VertiGIS Studio Workflow.
This article will walk you through creating a form element that uses the qrcode library to generate and display a QR code.
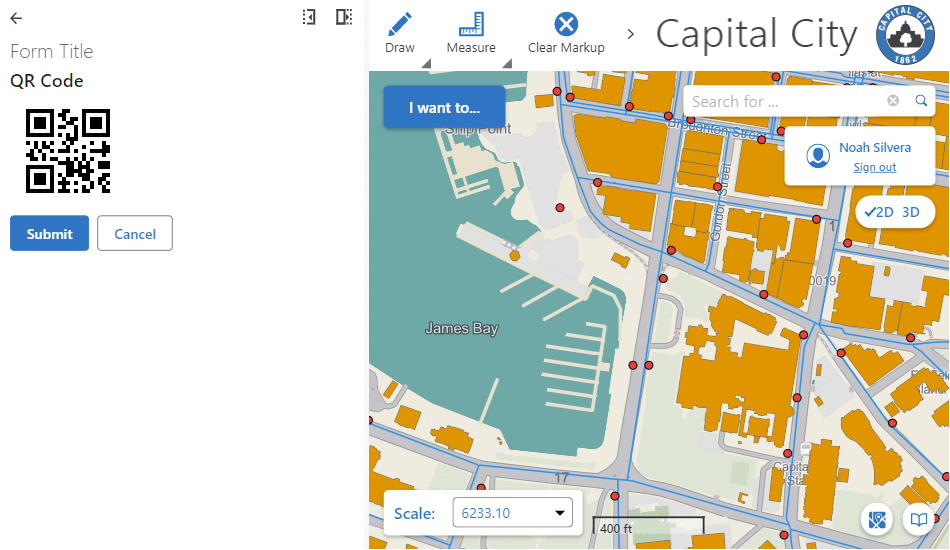
Prerequisites
Follow the instructions in the Web Applications SDK page to set up your development environment.
A working knowledge of TypeScript is recommended before extending Workflow for web-based hosts.
Overview
Implementing a QR code custom form element for web applications using a third party library consists of the following steps:
- Adding third party library dependencies to your project.
- Creating the custom form element.
- Implementing the custom form element using the third party library.
Adding Dependencies
- Run
npm install qrcode
in the terminal to install the third party qrcode library that generates QR codes. - Run
npm install @types/qrcode
in the terminal to install type information for the third party library. This is optional, but it provides an improved developer experience.
Set up Custom Form Element Skeleton
To create a new form element:
- Open the Workflow activity SDK in Visual Studio Code.
- Run
npm run generate
in the terminal. - When prompted, select
Form Element
. - Enter the name of the form element you would like to create and press
Enter
. For example,QrCode
. - Open the newly created
src/elements/QrCode.tsx
file.
Implement the Custom Form Element
Modify the skeleton form element implementation in src/elements/QrCode.tsx
to match the following example.
import * as React from "react";
import { useEffect, useState } from "react";
import QRCode from "qrcode";
import {
FormElementProps,
FormElementRegistration,
} from "@vertigis/workflow";
interface QrCodeProps extends FormElementProps<string> {}
function QrCode(props: QrCodeProps): React.ReactElement | null {
const { value } = props;
const [qrCodeUrl, setQrCodeUrl] = useState("");
// Update the QR code when the value changes
useEffect(() => {
// Clear the previous QR code
setQrCodeUrl("");
if (value) {
// Asynchronously generate the QR code data URL from the value
let didCancel = false;
(async () => {
const dataUrl = await QRCode.toDataURL(value);
if (didCancel) {
return;
}
setQrCodeUrl(dataUrl);
})();
return () => {
didCancel = true;
};
}
}, [value]);
// Render the QR code as an image
return qrCodeUrl ? <img src={qrCodeUrl} /> : null;
}
const QrCodeElementRegistration: FormElementRegistration<QrCodeProps> =
{
component: QrCode,
getInitialProperties: () => ({ value: "" }),
id: "QrCode",
};
export default QrCodeElementRegistration;
Deploy the Form Element
Follow the instructions to build and deploy the activity pack.
Test the Form Element
Once your activity pack is hosted and registered, your QrCode
custom form element should appear in the form element toolbox in VertiGIS Studio Workflow Designer alongside the built-in form elements.
To use your custom form element in a workflow:
- Select the
Display Form
activity that you want to include your form element in. - Locate the
QrCode
form element in the toolbox and drag it onto the form. - Alternatively:
- Add a
Custom
form element to the form. - Set the
Custom Type
property of theCustom
form element toQrCode
.
- Add a
- Add a
load
event subworkflow to the form element. - Add a
Set Form Element Property
activity to the subworkflow. - Set the
Property Name
input tovalue
. - Set the
Property Value
input to the text that represents the data or URL to encode into the QR code. - Test your workflow.
Next Steps
Learn how to create a QR Code activity
Learn how to to use this same third party library to create an activity that creates QR codes