Create an Activity
This article will walk you through creating a new workflow activity for VertiGIS Studio Desktop applications.
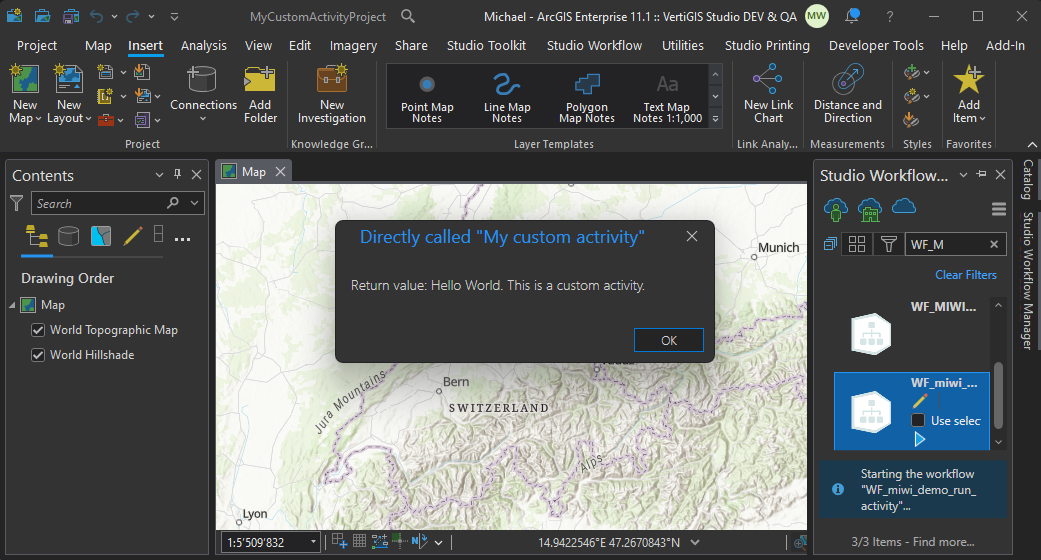
Prerequisites
While extending VertiGIS Studio Workflow for Desktop can easily be achieved, you require an installed Workflow runtime module to make your custom activities work with VertiGIS Studio Desktop.
To ease using your custom activities when authoring of Workflows with Workflow Designer, you may create method stubs for your activities and publish them to make them, as described in the corresponding chapter.
A working knowledge of C# and .NET Standard is recommended before extending Workflow for VertiGIS Studio Desktop
Create the Activity
- If you haven't done yet, create a new Visual Studio project using the
ArcGIS Pro Module Add-In
project template. - Create a new file
MyCustomActivity.cs
in the previously mentioned project. - Add a new skeleton workflow activity that implements
IActivityHandler
.
using System.Collections.Generic;
using System.Threading.Tasks;
using VertiGIS.Workflow.Runtime;
[assembly:WorkflowActivities]
namespace Poc_ProCustomActivities
{
public class MyCustomActivity : IActivityHandler
{
public static string Action => "uuid:cc39c481-4d05-4c39-8363-07b79dc03aa7::MyCustomActivity";
public Task<IDictionary<string, object?>> Execute(IDictionary<string, object?> inputs, IActivityContext context)
{
IDictionary<string, object?> outputs = new Dictionary<string, object?>();
outputs["test"] = "Hello World. This is a custom activity.";
return Task.FromResult(outputs);
}
}
}
It's important to conform to two necessary constraints to make the activity recognizable to VertiGIS Studio Desktop:
- Inside your assembly you need to add the
WorkflowActivities
attribute (make sure to add it only once across your whole project, e.g. in your module rather than inside your activity) - Your custom activity needs to contain an action identifier that tells VertiGIS Studio Desktop what activity is represented by this implementation. This can be achieved by declaring a static string property named
Action
that returns the activities action id. As static members cannot be specified in interfaces, you have to ensure this property being present yourself.
Ignoring any of those rules will lead to the activity not being found by VertiGIS Studio Desktop and thus cause a runtime error.
Use the Activity in a Workflow
Workflows that run in your custom VertiGIS Studio Desktop application can now run this custom activity.
Registering stubs for .NET activities provides a user friendly interface for your custom activities in VertiGIS Studio Workflow Designer.
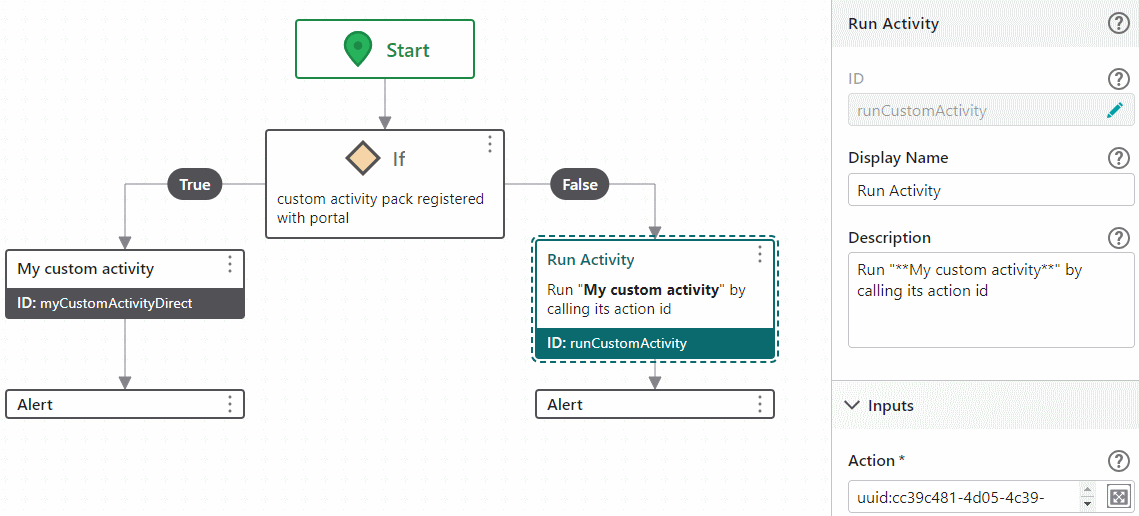
Without registering stubs you may still call your custom activity using the RunActivity
by the name defined in MyCustomActivity.cs
(for this example, uuid:cc39c481-4d05-4c39-8363-07b79dc03aa7::MyCustomActivity
).
You can
download this demo workflow
that runs the custom activity and
import it into the VertiGIS Studio Workflow Designer.
Next you need to run the workflow you just created in your VertiGIS Studio Desktop project.
https://apps.vertigisstudio.com/workflow/designer/#workflow= 44010fc421dd4659b74fb921e09ba594