Create a Service
This tutorial assumes you are using windows and can run the Universal Windows Version of VertiGIS Studio Mobile.
Sometimes, you may have logic or data that needs to be shared by multiple components across an app. In VertiGIS Studio Mobile, services are shared singletons that can register global commands and operations, be injected into components, run background tasks, and more.
Prerequisites
Check out and setup the VertiGIS Studio Mobile SDK Quickstart project.
Basic Service
Create a new file services/CustomService.cs
under the platform agnostic project.
In the file, add a new service class CustomService
and register it with VertiGIS Studio Mobile using an assembly attribute.
using App1.Services;
using VertiGIS.Mobile.Composition;
using VertiGIS.Mobile.Composition.Services;
using System.Threading.Tasks;
[assembly: Service(typeof(CustomService))]
namespace App1.Services
{
class CustomService : ServiceBase
{
public CustomService()
:base()
{
}
}
}
Create a Custom Command
Custom Services can register custom commands and operations. The following example shows how a custom service can register a command with the Operations Registry
, and how that command can be configured to run on a button press using layout and app config.
- Service
- Layout
- App Config
- User Interface
using App1.Services;
using VertiGIS.Mobile.Composition;
using VertiGIS.Mobile.Composition.Messaging;
using VertiGIS.Mobile.Composition.Services;
using System.Threading.Tasks;
[assembly: Service(typeof(CustomService))]
namespace App1.Services
{
public class CustomService : ServiceBase
{
public CustomService(IOperationRegistry operationRegistry)
: base()
{
operationRegistry.VoidOperation<string>("custom.alert").RegisterExecute((string customMessage) =>
{
return Application.Current.MainPage.DisplayAlert("Custom Alert", customMessage, "Cancel");
}, this);
}
}
}
<?xml version="1.0" encoding="utf-8" ?>
<layout
xmlns="https://geocortex.com/layout/v1"
xmlns:gxm="https://geocortex.com/layout/mobile/v1"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://geocortex.com/layout/v1 ../../ViewerSpec/layout/layout-mobile.xsd">
<map id="map1" >
<button id="custom-alert" config="custom-alert" style="map-widget" show-title="true" margin="0.3" slot="top-center"/>
</map>
</layout>
{
"$schema": "..\\..\\ViewerFramework\\app-config\\mobile\\mobile-app-config.schema.json",
"schemaVersion": "1.0",
"items": [
{
"id": "desktop-layout",
"$type": "layout",
"url": "resource://layout-large.xml",
"tags": ["medium", "large"]
},
{
"id": "custom-alert",
"iconId": "warning",
"title": "Custom Alert",
"action": {
"name": "custom.alert",
"arguments": "My Custom Message"
},
"$type": "menu-item"
}
]
}
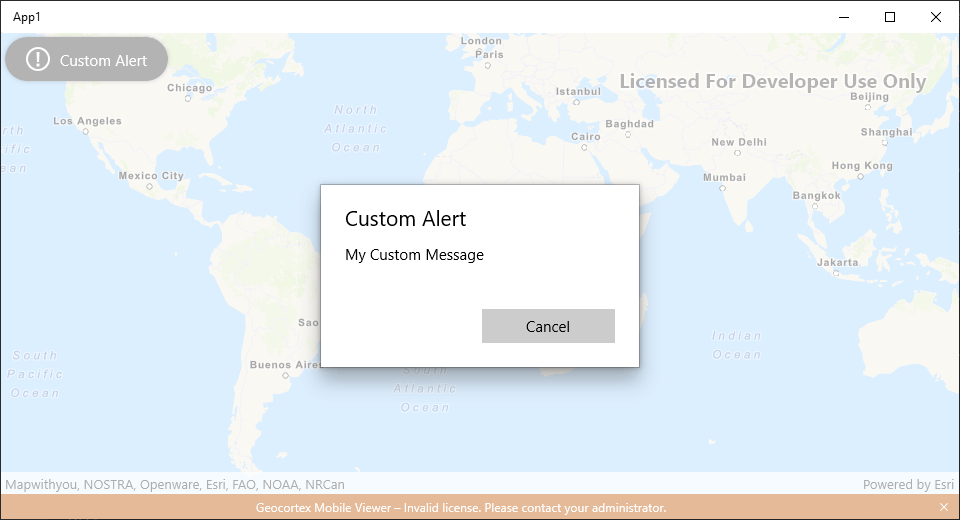
Relevant SDK Samples
Check out the relevant VertiGIS Studio Mobile SDK Samples:
Next Steps
Learn More About Services
Take a deep dive into services in the VertiGIS Studio Mobile SDK
Build a Service that Manages Dynamic Data
Built a service that manages a dynamic data source and exposes it to components