Create an Activity
This article will walk you through creating a new workflow activity for VertiGIS Studio Mobile applications.
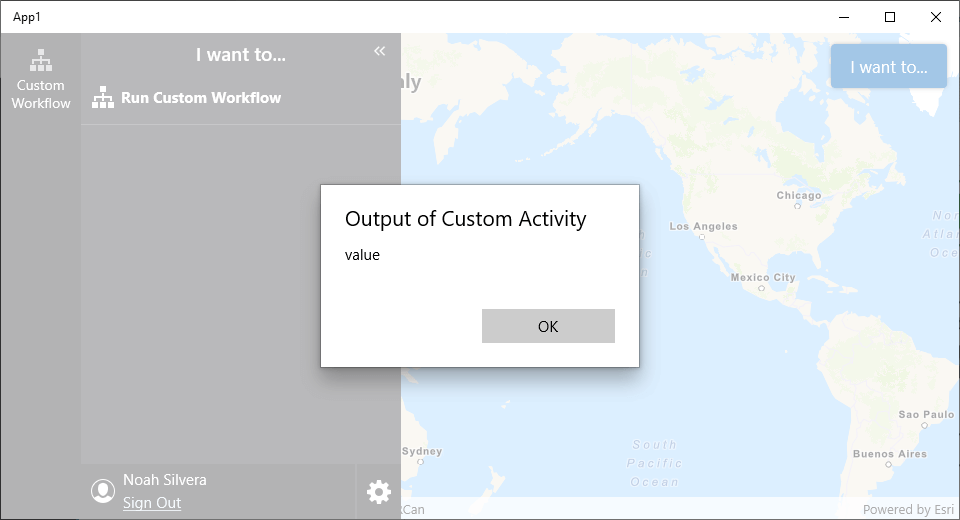
Prerequisites
Extending VertiGIS Studio Workflow for Mobile requires development and deployment of a custom VertiGIS Studio Mobile Application using the VertiGIS Studio Mobile SDK
Follow the instructions in the VertiGIS Studio Mobile SDK page to set up the environment for extending Workflow for VertiGIS Studio Mobile.
A working knowledge of C# and .NET Standard is recommended before extending Workflow for VertiGIS Studio Mobile
Create the Activity
- Create a new file
CustomActivity.cs
in the platform agnostic project of the VertiGIS Studio Mobile Quickstart. - Add a new skeleton workflow activity that implements
IActivityHandler
.
using App1.Workflow;
using VertiGIS.Mobile.Composition;
using VertiGIS.Workflow.Runtime;
using System.Collections.Generic;
using System.Threading.Tasks;
[assembly: Export(typeof(CustomActivity))]
namespace App1.Workflow
{
public class CustomActivity : IActivityHandler
{
public static string Action { get; } = "uuid:<uuid>::CustomActivity";
public Task<IDictionary<string, object>> Execute(IDictionary<string, object> inputs, IActivityContext context)
{
return Task.FromResult((IDictionary<string, object>)new Dictionary<string, object>(){
["test"] = "value"
});
}
}
}
Register the Activity with the IActivityHandlerFactory
- Create a new file,
ActivityLoader.cs
in the platform agnostic project of the VertiGIS Studio Mobile Quickstart. - Implement the
IActivityHandlerFactory
interface and register the activity skeleton we created in the constructor.
using System;
using System.Collections.Generic;
using System.Threading;
using System.Threading.Tasks;
using VertiGIS.Mobile.Composition;
using VertiGIS.Workflow.Runtime;
using VertiGIS.Workflow.Runtime.Definition;
using VertiGIS.Workflow.Runtime.Execution;
using App1.Workflow;
[assembly: Export(typeof(ActivityLoader), SingleInstance = true, AsImplementedInterfaces = true)]
namespace App1.Workflow
{
public class ActivityLoader : IActivityHandlerFactory
{
/// <summary>
/// Gets a mapping of action names to implementations of <see cref="IActivityHandler"/>s.
/// </summary>
private Dictionary<string, Func<IActivityHandler>> RegisteredActivities { get; } = new Dictionary<string, Func<IActivityHandler>>();
public ActivityLoader(Func<CustomActivity> customActivityFactory)
{
RegisteredActivities[CustomActivity.Action] = customActivityFactory;
}
/// <summary>
/// Creates an <see cref="IActivityHandler"/>.
/// </summary>
/// <param name="action">The name of the action to create.</param>
/// <param name="token">The cancellation token.</param>
/// <param name="inspector">The <see cref="ProgramInspector"/> for the program.</param>
/// <returns>The activity handler for the given action.</returns>
public Task<IActivityHandler> Create(string action, CancellationToken token, ProgramInspector inspector = null)
{
if (action == null || token.IsCancellationRequested)
{
return Task.FromResult<IActivityHandler>(null);
}
if (RegisteredActivities.TryGetValue(action, out Func<IActivityHandler> handlerType))
{
return Task.FromResult(handlerType());
}
else
{
return Task.FromResult<IActivityHandler>(null);
}
}
}
}
Use the Activity in a Workflow
Workflows that run in your custom VertiGIS Studio Mobile application can now run this custom activity.
Registering stubs for .NET activities provides a user friendly interface for your custom activities in VertiGIS Studio Workflow Designer.
The RunActivity
activity can be used to execute your activity by the name defined in CustomActivity.cs
(for this example, uuid:<uuid>::CustomActivity
).
You can
download this demo workflow
that runs the custom activity and
import it into the VertiGIS Studio Workflow Designer.
Next you need to run the workflow you just created in your VertiGIS Studio Mobile SDK project.
You can do this by configuring the layout and app config to run a workflow. You will need to copy the ID of the the workflow you created into the app.json
https://apps.vertigisstudio.com/workflow/designer/#workflow= 44010fc421dd4659b74fb921e09ba594
- App Config
- Layout
- UI
{
"schemaVersion": "1.0",
"items": [
{
"$type": "layout",
"id": "desktop-layout",
"url": "resource://layout-large.xml",
"tags": ["large"]
},
{
"$type": "workflow",
"id": "custom-workflow",
"title": "Custom Workflow",
"target": "#taskbar",
"portalItem": "<your-workflow-id>"
},
{
"$type": "menu",
"id": "iwtm",
"items": [
{
"title": "Run Custom Workflow",
"isEnabled": true,
"iconId": "workflow",
"action": {
"name": "workflow.run",
"arguments": {
"id": "custom-workflow"
}
}
}
]
}
]
}
<?xml version="1.0" encoding="utf-8" ?>
<layout
xmlns="https://geocortex.com/layout/v1"
xmlns:gxm="https://geocortex.com/layout/mobile/v1"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://geocortex.com/layout/v1 ../../ViewerSpec/layout/layout-mobile.xsd">
<gxm:taskbar id="taskbar" orientation="vertical">
<map slot="main">
<stack margin="0.8" slot="top-right" halign="end">
<iwtm config="iwtm"/>
</stack>
</map>
</gxm:taskbar>
</layout>
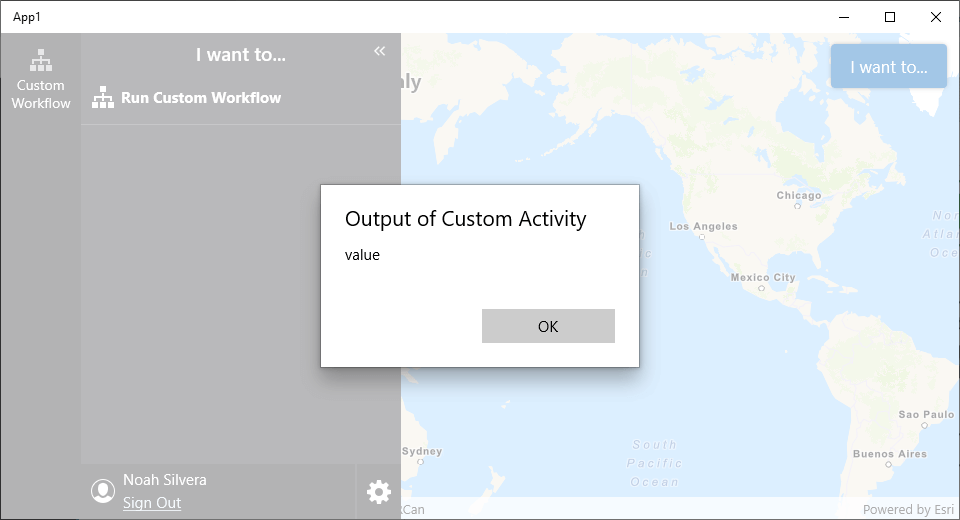
Next Steps
Calculate a Logarithm with a Custom Activity
Implement a custom activity that calculates the logarithm of a number
Implement a Custom Form Element
Implement a custom form element for applications like VertiGIS Studio Mobile
Add a Callout to the Map through a Custom Activity
Access the map in custom activities for VertiGIS Studio Mobile
Use the ArcGIS Runtime SDK for .NET in an activity.
Use the ArcGIS Runtime SDK for .NET in an activity or form element